Index
Styling React Components Elements
Button should have green background color when you click show persons, red background color if you have to remove the person list.const style = {
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer'
};
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer'
};
style.backgroundColor = ‘red’;
persons = (
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
style.backgroundColor = 'red';
}
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
style.backgroundColor = 'red';
}
Linked file: green-red.gif |
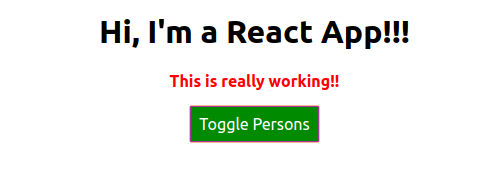
let classes = ['red', 'bold'].join(' ');
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
Setting className dynamically:
const classes = [];
if (this.state.persons.length <= 2) {
classes.push('red');
}
if (this.state.persons.length <= 1) {
classes.push('bold');
}
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
if (this.state.persons.length <= 2) {
classes.push('red');
}
if (this.state.persons.length <= 1) {
classes.push('bold');
}
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
Linked file: dynamic_css.gif |
Adding and Using Radium:
npm install --save radium
With using Radium you can pseudo-selector and media queries.
pseudo classes include :hover, :link, :visited, :active etc.
To use radium you should include it:
import React, { Component } from 'react';
import './App.css';
import Radium from 'radium';
import Person from './Person/Person';
import './App.css';
import Radium from 'radium';
import Person from './Person/Person';
export default Radium(App);
Now all pseudo classes are supported, but you have to wrap them in quotation marks, as it contain invalid character : to be named as property.
Change of color:, from green to MediumSeaGreen when hover and Tomato on click
const style = {
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer',
':hover': {
backgroundColor:'MediumSeaGreen',
color:'black',
}
};
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer',
':hover': {
backgroundColor:'MediumSeaGreen',
color:'black',
}
};
style.backgroundColor = 'red';
style[':hover'] = {
backgroundColor: 'Tomato',
color: 'black'
};
App.js (Complete code)
import React, { Component } from 'react';
import './App.css';
import Radium from 'radium';
import Person from './Person/Person';
class App extends Component {
state = {
persons: [
{ id: 'abc1', name: 'Max', age: 28},
{ id: 'abc2', name: 'Manu', age: 29},
{ id: 'abc3', name: 'Stephanie', age:26},
{ id: 'abc4', name: 'Amiya', age:31}
],
otherState: 'some other value',
showPersons: false
}
deletePersonHandler = (personIndex) => {
const persons = [...this.state.persons];
persons.splice(personIndex,1);
this.setState({persons:persons});
}
nameChangedHandler = (event, id) => {
const personIndex = this.state.persons.findIndex(p => {
return p.id === id;
});
const person = { ...this.state.persons[personIndex]
};
person.name = event.target.value;
const persons = [...this.state.persons];
persons[personIndex] = person;
this.setState({
persons: persons
});
}
togglePersonsHandler = () => {
const doesShow = this.state.showPersons;
this.setState({showPersons:!doesShow})
}
render() {
const style = {
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer',
':hover': {
backgroundColor:'MediumSeaGreen',
color:'black',
}
};
let persons = null;
if(this.state.showPersons){
persons = (
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
style.backgroundColor = 'red';
style[':hover'] = {
backgroundColor: 'Tomato',
color: 'black'
};
}
const classes = [];
if (this.state.persons.length <= 2) {
classes.push('red');
}
if (this.state.persons.length <= 1) {
classes.push('bold');
}
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
}
}
export default Radium(App);
import './App.css';
import Radium from 'radium';
import Person from './Person/Person';
class App extends Component {
state = {
persons: [
{ id: 'abc1', name: 'Max', age: 28},
{ id: 'abc2', name: 'Manu', age: 29},
{ id: 'abc3', name: 'Stephanie', age:26},
{ id: 'abc4', name: 'Amiya', age:31}
],
otherState: 'some other value',
showPersons: false
}
deletePersonHandler = (personIndex) => {
const persons = [...this.state.persons];
persons.splice(personIndex,1);
this.setState({persons:persons});
}
nameChangedHandler = (event, id) => {
const personIndex = this.state.persons.findIndex(p => {
return p.id === id;
});
const person = { ...this.state.persons[personIndex]
};
person.name = event.target.value;
const persons = [...this.state.persons];
persons[personIndex] = person;
this.setState({
persons: persons
});
}
togglePersonsHandler = () => {
const doesShow = this.state.showPersons;
this.setState({showPersons:!doesShow})
}
render() {
const style = {
backgroundColor: 'green',
color: 'white',
font: 'inherit',
border: '1px solid blue',
padding: '8px',
cursor:'pointer',
':hover': {
backgroundColor:'MediumSeaGreen',
color:'black',
}
};
let persons = null;
if(this.state.showPersons){
persons = (
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
style.backgroundColor = 'red';
style[':hover'] = {
backgroundColor: 'Tomato',
color: 'black'
};
}
const classes = [];
if (this.state.persons.length <= 2) {
classes.push('red');
}
if (this.state.persons.length <= 1) {
classes.push('bold');
}
return (
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
}
}
export default Radium(App);
Also include Radium on person.js
import React from 'react';
import Radium from 'radium';
import './Person.css';
const person = (props) => {
return (
<div className="Person">
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default Radium(person);
import Radium from 'radium';
import './Person.css';
const person = (props) => {
return (
<div className="Person">
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default Radium(person);
Using Radium for Media Queries
const style = {
'@media (min-width:500px)': {
width: '450px'
}
<div className="Person" style={style}>
import React from 'react';
import Radium from 'radium';
import './Person.css';
const person = (props) => {
const style = {
'@media (min-width:500px)': {
width: '450px'
}
};
return (
<div className="Person" style={style}>
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default Radium(person);
import Radium from 'radium';
import './Person.css';
const person = (props) => {
const style = {
'@media (min-width:500px)': {
width: '450px'
}
};
return (
<div className="Person" style={style}>
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default Radium(person);
Also you need to wrap the whole application with that:
App.js
import Radium, {StyleRoot} from 'radium';
<StyleRoot>
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
</StyleRoot>
<div className="App">
<h1>Hi, I'm a React App!!!</h1>
<p className={classes.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
</StyleRoot>
Using CSS Modules
To use css module, use the css extension as .module.css
import classes from './App.module.css';
const assignedClasses = [];
if (this.state.persons.length <= 2) {
assignedClasses.push(classes.red);
}
if (this.state.persons.length <= 1) {
assignedClasses.push(classes.bold);
}
return (
<div className={classes.App}>
<h1>Hi, I'm a React App!!!</h1>
<p className={ assignedClasses.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
if (this.state.persons.length <= 2) {
assignedClasses.push(classes.red);
}
if (this.state.persons.length <= 1) {
assignedClasses.push(classes.bold);
}
return (
<div className={classes.App}>
<h1>Hi, I'm a React App!!!</h1>
<p className={ assignedClasses.join(' ')}>This is really working!! </p>
<button style={style} onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
.App {
text-align: center;
}
.red {
color:red;
}
.bold {
font-weight: bold;
}
text-align: center;
}
.red {
color:red;
}
.bold {
font-weight: bold;
}
You can get css selectors as attribute,
here..
classes.App, classes.red, classes.bold
Adding Pseudo Selectors
App.module.css
.App {
text-align: center;
}
.red {
color:red;
}
.bold {
font-weight: bold;
}
.App button {
border: 1px solid blue;
padding: 16px;
background-color: green;
font: inherit;
color: white;
}
.App button:hover {
background-color: yellow;
color: black;
}
.App button.Red {
background-color: red;
}
.App button.Red:hover {
background-color: blue;
color: black;
}
text-align: center;
}
.red {
color:red;
}
.bold {
font-weight: bold;
}
.App button {
border: 1px solid blue;
padding: 16px;
background-color: green;
font: inherit;
color: white;
}
.App button:hover {
background-color: yellow;
color: black;
}
.App button.Red {
background-color: red;
}
.App button.Red:hover {
background-color: blue;
color: black;
}
btnClass = classes.Red;
<button
className={btnClass}
onClick={this.togglePersonsHandler}>Toggle Persons</button>
import React, { Component } from 'react';
import classes from './App.module.css';
import Person from './Person/Person';
class App extends Component {
state = {
persons: [
{ id: 'abc1', name: 'Max', age: 28},
{ id: 'abc2', name: 'Manu', age: 29},
{ id: 'abc3', name: 'Stephanie', age:26},
{ id: 'abc4', name: 'Amiya', age:31}
],
otherState: 'some other value',
showPersons: false
}
deletePersonHandler = (personIndex) => {
const persons = [...this.state.persons];
persons.splice(personIndex,1);
this.setState({persons:persons});
}
nameChangedHandler = (event, id) => {
const personIndex = this.state.persons.findIndex(p => {
return p.id === id;
});
const person = { ...this.state.persons[personIndex]
};
person.name = event.target.value;
const persons = [...this.state.persons];
persons[personIndex] = person;
this.setState({
persons: persons
});
}
togglePersonsHandler = () => {
const doesShow = this.state.showPersons;
this.setState({showPersons:!doesShow})
}
render() {
let persons = null;
let btnClass = '';
if(this.state.showPersons){
persons = (
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
btnClass = classes.Red;
}
const assignedClasses = [];
if (this.state.persons.length <= 2) {
assignedClasses.push(classes.red);
}
if (this.state.persons.length <= 1) {
assignedClasses.push(classes.bold);
}
return (
<div className={classes.App}>
<h1>Hi, I'm a React App!!!</h1>
<p className={ assignedClasses.join(' ')}>This is really working!! </p>
<button
className={btnClass}
onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
}
}
export default App;
import classes from './App.module.css';
import Person from './Person/Person';
class App extends Component {
state = {
persons: [
{ id: 'abc1', name: 'Max', age: 28},
{ id: 'abc2', name: 'Manu', age: 29},
{ id: 'abc3', name: 'Stephanie', age:26},
{ id: 'abc4', name: 'Amiya', age:31}
],
otherState: 'some other value',
showPersons: false
}
deletePersonHandler = (personIndex) => {
const persons = [...this.state.persons];
persons.splice(personIndex,1);
this.setState({persons:persons});
}
nameChangedHandler = (event, id) => {
const personIndex = this.state.persons.findIndex(p => {
return p.id === id;
});
const person = { ...this.state.persons[personIndex]
};
person.name = event.target.value;
const persons = [...this.state.persons];
persons[personIndex] = person;
this.setState({
persons: persons
});
}
togglePersonsHandler = () => {
const doesShow = this.state.showPersons;
this.setState({showPersons:!doesShow})
}
render() {
let persons = null;
let btnClass = '';
if(this.state.showPersons){
persons = (
<div>
{this.state.persons.map((person,index) => {
return <Person
click= {() => this.deletePersonHandler(index)}
name={person.name}
age = {person.age}
key = {person.id}
changed={(event) => this.nameChangedHandler(event, person.id)}/>
})}
</div>
);
btnClass = classes.Red;
}
const assignedClasses = [];
if (this.state.persons.length <= 2) {
assignedClasses.push(classes.red);
}
if (this.state.persons.length <= 1) {
assignedClasses.push(classes.bold);
}
return (
<div className={classes.App}>
<h1>Hi, I'm a React App!!!</h1>
<p className={ assignedClasses.join(' ')}>This is really working!! </p>
<button
className={btnClass}
onClick={this.togglePersonsHandler}>Toggle Persons</button>
{persons}
</div>
);
}
}
export default App;
Linked file: cssmodule_hover.gif |
Working with Media Queries
Person.js
import React from 'react';
import classes from './Person.module.css';
const person = (props) => {
return (
<div className={classes.Person}>
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default person;
import classes from './Person.module.css';
const person = (props) => {
return (
<div className={classes.Person}>
<p onClick={props.click}>I'm {props.name} and I am {props.age} years old!</p>
<p>{props.children}</p>
<input type="text" onChange={props.changed} value={props.name} />
</div>
);
};
export default person;
Person.module.css
.Person {
margin: 16px auto;
border: 1px solid #eee;
box-shadow: 0 2px 3px #ccc;
padding: 16px;
text-align: center;
}
@media (min-width:500px) {
.Person {
width: 400px;
}
}
margin: 16px auto;
border: 1px solid #eee;
box-shadow: 0 2px 3px #ccc;
padding: 16px;
text-align: center;
}
@media (min-width:500px) {
.Person {
width: 400px;
}
}