Index
Arrow Function
Arrow Function:const printMyName = (name) => {
console.log(name);
}
printMyName('Max');
console.log(name);
}
printMyName('Max');
For single argument, it can also be written as:
const printMyName = name => {
console.log(name)
}
printMyName('Max');
console.log(name)
}
printMyName('Max');
For no argument:
const printMyName = () => {
console.log("Max);
}
console.log("Max);
}
Exports and Imports (Modules)
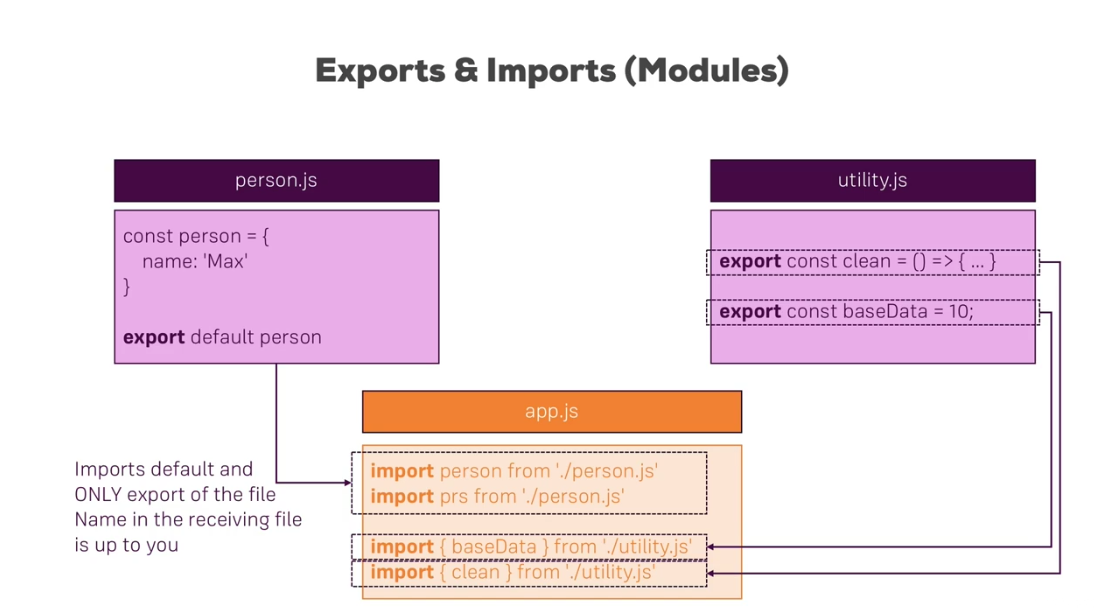
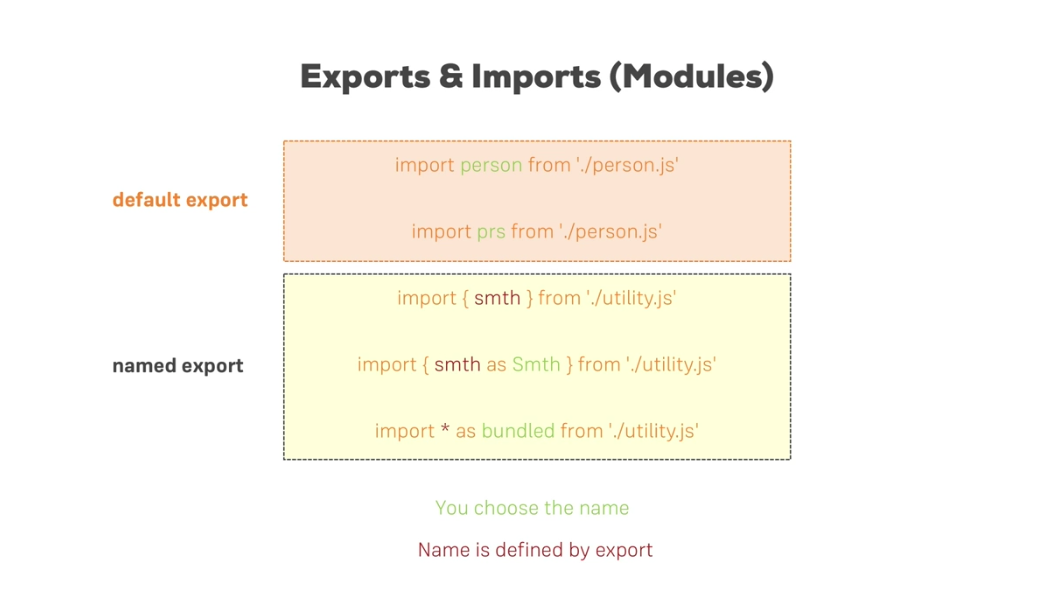
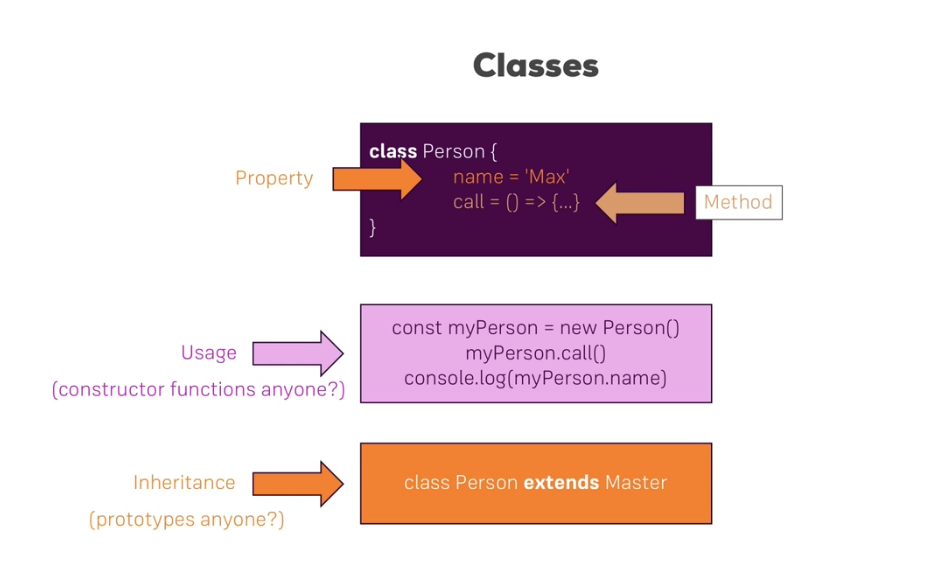
class Person {
constructor () {
this.name = 'Max';
}
printMyName(){
console.log(this.name);
}
}
const person = new Person();
person.printMyName();
constructor () {
this.name = 'Max';
}
printMyName(){
console.log(this.name);
}
}
const person = new Person();
person.printMyName();
class Human {
constructor() {
this.gender = 'male';
}
printGender(){
console.log(this.gender);
}
}
class Person {
constructor () {
super();
this.name = 'Max';
this.gender = 'female';
}
printMyName(){
console.log(this.name);
}
}
const person = new Person();
person.printMyName();
person.printGender();
constructor() {
this.gender = 'male';
}
printGender(){
console.log(this.gender);
}
}
class Person {
constructor () {
super();
this.name = 'Max';
this.gender = 'female';
}
printMyName(){
console.log(this.name);
}
}
const person = new Person();
person.printMyName();
person.printGender();
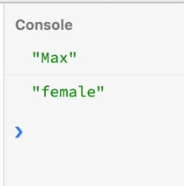