Election
pragma solidity >=0.4.22 <0.7.0;
contract Election {
// Model a candidate
struct Candidate {
uint id;
string name;
uint voteCount;
}
// Store candidate
// Fetch Candidate
mapping(uint => Candidate) public candidates;
// Store candidate count
uint public candidatesCount;
constructor () public {
addCandidate("Candidate 1");
addCandidate("Candidate 2");
}
function addCandidate(string memory _name) private {
candidatesCount ++;
candidates[candidatesCount] = Candidate(candidatesCount, _name, 0);
}
}
contract Election {
// Model a candidate
struct Candidate {
uint id;
string name;
uint voteCount;
}
// Store candidate
// Fetch Candidate
mapping(uint => Candidate) public candidates;
// Store candidate count
uint public candidatesCount;
constructor () public {
addCandidate("Candidate 1");
addCandidate("Candidate 2");
}
function addCandidate(string memory _name) private {
candidatesCount ++;
candidates[candidatesCount] = Candidate(candidatesCount, _name, 0);
}
}
// Store candidate
// Fetch Candidate
mapping(uint => Candidate) public candidates;
// Fetch Candidate
mapping(uint => Candidate) public candidates;
Mapping in solidity is like an associative array where we associate key value pairs.
We declare this mapping with a mapping keyword, then we pass in the types that we expect for each key and value pair.
In this case, key will be an unsigned integer, which corresponds to candidate id, and value will be a candidate structure type.
This is the way we will store our candidates, we can declare this mapping public, and solidity will generate a candidate function for us that allow us to fetch our candidate from mapping.
When we add a candidate to our mapping, we are changing the state of the contract, and writing to the blockchain.
We need a keep track of how many candidates we have created, in our election, and I am gonna do this with a countercash,
// Store candidate count
uint public candidatesCount;
uint public candidatesCount;
In solidity there is no way to determine size of the mapping, also no way to iterate over it either.
If you have two candidates in election, and you look up for candidate number 99, the value will return a blank candidate.
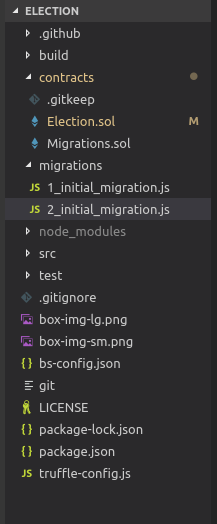
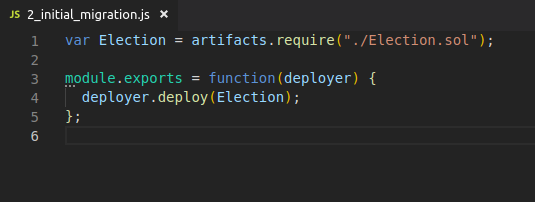
var Election = artifacts.require("./Election.sol");
module.exports = function(deployer) {
deployer.deploy(Election);
};
module.exports = function(deployer) {
deployer.deploy(Election);
};
truffle migrate
truffle console
Election.deployed().then(function(instance) { app = instance} )
app.address
'0xB929B577ab8cb59B28d396fD8Ac559cb62001785'
Election.deployed().then(function(instance) { app = instance} )
app.address
'0xB929B577ab8cb59B28d396fD8Ac559cb62001785'
After code changes
truffle migrate --reset
truffle console
Election.deployed().then(function(instance) { app = instance} )
Election.deployed().then(function(instance) { app = instance} )
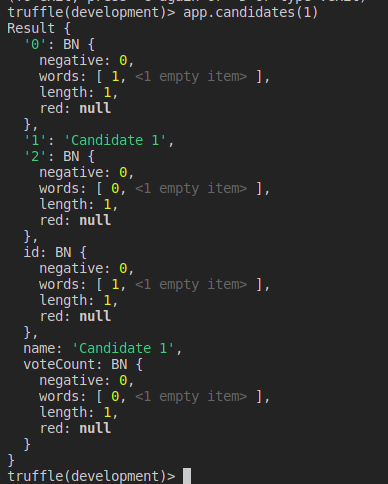
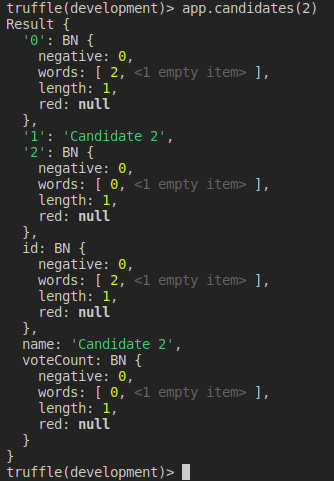

app.candidates(1).then(function(c) { candidate = c; })
candidate
candidate
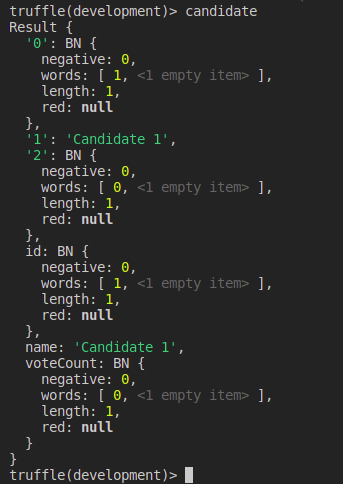
Access id, name, voteCount
See the value in words.

toNumber()
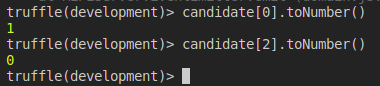